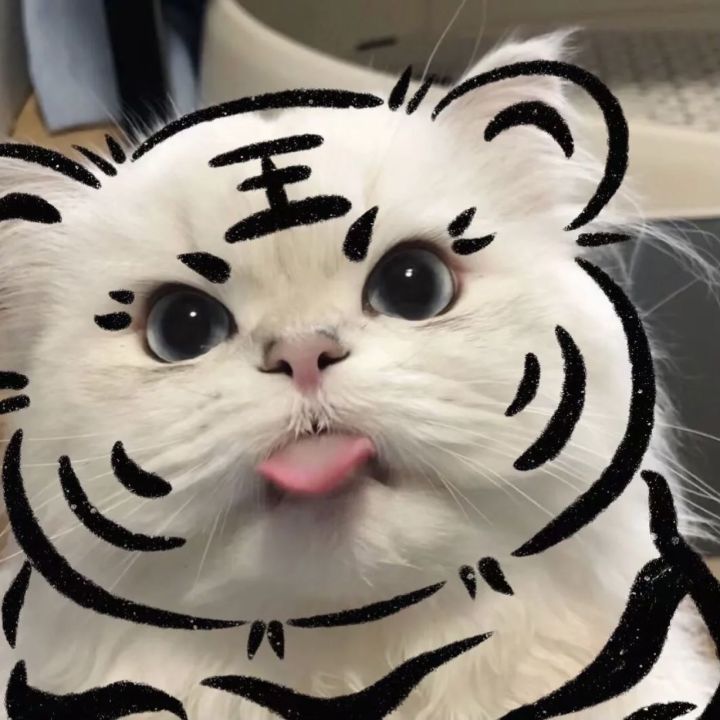
主 题
for、while循环和递归中async/await的使用
// 公共代码
const timeOut = (item, time=1000) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(item);
}, time);
});
};
const dataArr = [1, 2, 3, 4, 5];
for
const forTest = async () => {
for (let i = 0; i < dataArr.length; i++) {
const res = await timeOut(dataArr[i])
console.log(res)
}
}
forTest() // 同步依次输出 1 2 3 4 5
for of
const forofTest = async () => {
for (const item of dataArr) {
const res = await timeOut(item)
console.log(res)
}
}
forofTest() // 同步依次输出 1 2 3 4 5
for in
const forinTest = async () => {
for (const item in dataArr) {
const res = await timeOut(dataArr[item])
console.log(res)
}
}
forinTest() // 同步依次输出 1 2 3 4 5
for await of
// 数组就是一个可迭代对象
const asyncIterable = [timeOut(2, 2000), timeOut(3, 3000), timeOut(1, 1000)]
const forAwaitOfTest = async () => {
// 异步迭代
for await (const item of asyncIterable) {
console.log(item)
}
}
forAwaitOfTest()// 同步依次输出 2 3 1
while
const whileTest = async () => {
let i = 0
while (i < dataArr.length) {
const res = await timeOut(dataArr[i++])
console.log(res)
}
}
whileTest() // 同步依次输出 1 2 3 4 5
递归
const diguiFunc = async (index = 0) => {
if (index < dataArr.length) {
const res = await timeOut(dataArr[index])
console.log(res)
index++
diguiFunc(index)
}
}
diguiFunc() // 同步依次输出 1 2 3 4 5
forEach
const foreachTest = async () => {
dataArr.forEach(async (item, index) => {
const res = await timeOut(dataArr[index])
console.log(res)
})
}
foreachTest() // 异步独立 同步一次输出 1 2 3 4 5
map类函数
const mapTest = async () => {
await Promise.all(
dataArr.map(async (item, index) => {
console.log(dataArr[index])
const res = await timeOut(dataArr[index])
console.log(res)
})
)
}
mapTest() // 异步独立同步一次输出 1 2 3 4 5
总结
for
,for of
,for in
,for await of
,while
和递归
中使用async/await
请求异步数据时,会同步依次执行循环体{代码}
里面的代码,即上一个await执行完再到下一个;
forEach
和map
类的函数使用传入回调函数的数组方法中使用async/await
,await
前的代码会立即执行。await
后的代码会再同步执行完之后一起执行,即每个异步的代码都是独立的,之间没有联系。
应用场景
- for循环类一般需要依次请求数据组装一个数组时用到(依次发请求请求);列:当页面多个图表时,可以使用到此类方法,按照数组顺序依次请求数据完成渲染图表。
- 当然不需要依赖上一个时,也可以组合多个
Promise
然后使用Promise.all([promise])
统一异步执行。
全部评论(0)